Verilog code for D Flip Flop
源自於https://www.fpga4student.com/2017/02/verilog-code-for-d-flip-flop.html
D Flip-Flop is a fundamental component in digital logic circuits. Verilog code for D Flip Flop is presented in this project. There are two types of D Flip-Flops being implemented which are Rising-Edge D Flip Flop and Falling-Edge D Flip Flop.
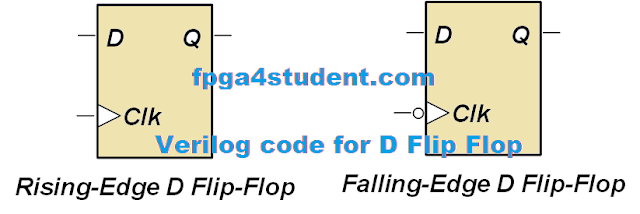
Verilog code for Rising Edge D Flip Flop:
// FPGA projects using Verilog/ VHDL
// fpga4student.com
// Verilog code for D Flip FLop
// Verilog code for rising edge D flip flop
module RisingEdge_DFlipFlop(D,clk,Q);
input D; // Data input
input clk; // clock input
output Q; // output Q
always @(posedge clk)
begin
Q <= D;
end
endmodule
Verilog code for Rising Edge D Flip-Flop with Synchronous Reset:
// FPGA projects using Verilog/ VHDL
// fpga4student.com
// Verilog code for D Flip FLop
// Verilog code for Rising edge D flip flop with Synchronous Reset input
module RisingEdge_DFlipFlop_SyncReset(D,clk,sync_reset,Q);
input D; // Data input
input clk; // clock input
input sync_reset; // synchronous reset
output reg Q; // output Q
always @(posedge clk)
begin
if(sync_reset==1'b1)
Q <= 1'b0;
else
Q <= D;
end
endmodule
Verilog code for Rising Edge D Flip-Flop with Asynchronous Reset High Level:
// FPGA projects using Verilog/ VHDL
// fpga4student.com
// Verilog code for D Flip FLop
// Verilog code for Rising edge D flip flop with Asynchronous Reset high
module RisingEdge_DFlipFlop_AsyncResetHigh(D,clk,async_reset,Q);
input D; // Data input
input clk; // clock input
input async_reset; // asynchronous reset high level
output reg Q; // output Q
always @(posedge clk or posedge async_reset)
begin
if(async_reset==1'b1)
Q <= 1'b0;
else
Q <= D;
end
endmodule
Verilog code for Rising Edge D Flip-Flop with Asynchronous Reset Low Level:
// FPGA projects using Verilog/ VHDL
// fpga4student.com
// Verilog code for D Flip FLop
// Verilog code for Rising edge D flip flop with Asynchronous Reset Low
module RisingEdge_DFlipFlop_AsyncResetLow(D,clk,async_reset,Q);
input D; // Data input
input clk; // clock input
input async_reset; // asynchronous reset low level
output reg Q; // output Q
always @(posedge clk or negedge async_reset)
begin
if(async_reset==1'b0)
Q <= 1'b0;
else
Q <= D;
end
endmodule
Verilog code for Falling Edge D Flip Flop:
// FPGA projects using Verilog/ VHDL
// fpga4student.com
// Verilog code for D Flip FLop
// Verilog code for falling edge D flip flop
module FallingEdge_DFlipFlop(D,clk,Q);
input D; // Data input
input clk; // clock input
output reg Q; // output Q
always @(negedge clk)
begin
Q <= D;
end
endmodule
Verilog code for Falling Edge D Flip-Flop with Synchronous Reset:
// FPGA projects using Verilog/ VHDL
// fpga4student.com
// Verilog code for D Flip FLop
// Verilog code for Falling edge D flip flop with Synchronous Reset input
module FallingEdge_DFlipFlop_SyncReset(D,clk,sync_reset,Q);
input D; // Data input
input clk; // clock input
input sync_reset; // synchronous reset
output reg Q; // output Q
always @(negedge clk)
begin
if(sync_reset==1'b1)
Q <= 1'b0;
else
Q <= D;
end
endmodule
Verilog code for Falling Edge D Flip-Flop with Asynchronous Reset High Level:
// FPGA projects using Verilog/ VHDL
// fpga4student.com
// Verilog code for D Flip FLop
// Verilog code for Falling edge D flip flop with Asynchronous Reset high
module FallingEdge_DFlipFlop_AsyncResetHigh(D,clk,async_reset,Q);
input D; // Data input
input clk; // clock input
input async_reset; // asynchronous reset high level
output reg Q; // output Q
always @(negedge clk or posedge async_reset)
begin
if(async_reset==1'b1)
Q <= 1'b0;
else
Q <= D;
end
endmodule
Verilog code for Falling Edge D Flip-Flop with Asynchronous Reset Low Level:
// FPGA projects using Verilog/ VHDL
// fpga4student.com
// Verilog code for D Flip FLop
// Verilog code for Falling edge D flip flop with Asynchronous Reset low
module FallingEdge_DFlipFlop_AsyncResetLow(D,clk,async_reset,Q);
input D; // Data input
input clk; // clock input
input async_reset; // asynchronous reset low level
output reg Q; // output Q
always @(negedge clk or negedge async_reset)
begin
if(async_reset==1'b0)
Q <= 1'b0;
else
Q <= D;
end
endmodule
Verilog Testbench code to simulate and verify D Flip-Flop:
`timescale 1ns/1ps;
// FPGA projects using Verilog/ VHDL
// fpga4student.com
// Verilog code for D Flip FLop
// Testbench Verilog code for verification
module tb_DFF();
reg D;
reg clk;
reg reset;
wire Q;
RisingEdge_DFlipFlop_SyncReset dut(D,clk,reset,Q);
initial begin
clk=0;
forever #10 clk = ~clk;
end
initial begin
reset=1;
D <= 0;
#100;
reset=0;
D <= 1;
#100;
D <= 0;
#100;
D <= 1;
end
endmodule
沒有留言:
張貼留言