There a several ways that you can copy nodes and flows between flows in the node-red Workspace.
They are:
- Select, Copy and Paste
- Export and Import Clipboard
- Save To and Retrieve from the Local Library
Copy and Paste
This works just like copying text in a word processor. You simply select the nodes you want using the CTRL key to select individual nodes together into a group or CTRL+A to select all nodes in the workspace.
Then use CTRL+C to Copy.
You then move to the place on the workspace or open the workspace where you want to copy the nodes to and use CTRL+V to paste the nodes/flow into the workspace.
Note: This only works for flows in the same workspace.
Using Export and Import and The Clipboard
This method can be used for exchanging flows between systems as well as on the same system.
To do this you need to highlight each node in the flow and then click onMenu>export>clipboard.
Note: Use CTRL+A to select all nodes in the workspace.
The contents of the export file will be displayed click on export to clipboard.
You then need to open a file editor and paste the contents into the file using CTRL+V.
Give the file a name and save it.
Importing A Flow
You will need to open the flow in a text editor, and then copy its contents usingCRTL+C.
You then go to Menu>import>clipboard and paste the file into the window and click the import button to complete the import.
Import Problems
If you find that the import button doesn’t change colour and a red line appears around the text box it is probably because what you are trying to import isn’t valid JSON.
You can test it by pasting it into this online JSON checker
A confusing issue I found is that my export file verified OK using the JSON checker but failed when doing an import at a remote location.
The problem was due to smart quotes in the export file.
智能引號清除工具
smart quotes removal tool
Using The Library
If you want to save a flow or function and reuse it in another flow then you can save it to the local library.
To save a flow to the library highlight all nodes in the flow by using CTRL+A then use the main menu (top right) and select Export>Library.
You will need to give the flow a name and optionally you can create a folder for the flow.
For example you could store flows in the flows folder and functions in the functions folder.
All flows are stored in the lib folder under the users .node-red folder.
To save a function to the library double click on the function to edit it and click on the bookmark icon next to the function name.
A drop down menu appears to either import or save the function to the library.
You need to give the file a name and optionally a folder to store it in.
Note: The function itself should have a name as you will need this when importing the function.
If you don’t give the function a name it appears as an unnamed function when importing.
Using Library Functions and Flows
To use a library flow use the Import>Library option and select the flow, and it appears in the flow canvas
To use a library function drag a function node into the flow canvas then open it for editing.
Click on the bookmark icon next to the function name and select open library from the drop down menu.
Open folder containing functions and the functions appear by name. Select the function and click load, to load the library code into the function.
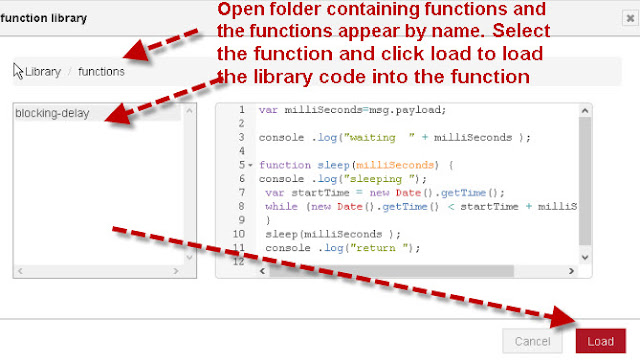
原始檔案
[{"id":"6aee26ac.b75e48","type":"inject","z":"afe7af4b.c2261","name":"","topic":"test","payload":"test message","payloadType":"str","repeat":"","crontab":"","once":false,"onceDelay":0.1,"x":140,"y":1700,"wires":[["b73ed76d.7bd3b8"]]},{"id":"b73ed76d.7bd3b8","type":"function","z":"afe7af4b.c2261","name":"message array","func":"var m_out=[]; //rray for message objects\nvar message=msg.payload;\nfor (i=0;i<3;i++){\n \n message=message+i; //add count to message\n var newmsg={payload:message,topic:msg.topic}\n m_out.push(newmsg);\n}\nreturn[m_out];\n","outputs":1,"noerr":0,"x":340,"y":1700,"wires":[["549f2f6c.0cc2a"]]},{"id":"549f2f6c.0cc2a","type":"debug","z":"afe7af4b.c2261","name":"","active":true,"tosidebar":true,"console":false,"tostatus":false,"complete":"false","x":530,"y":1700,"wires":[]}]
[{"id":"6aee26ac.b75e48","type":"inject","z":"afe7af4b.c2261","name":"","topic":"test","payload":"test message","payloadType":"str","repeat":"","crontab":"","once":false,"onceDelay":0.1,"x":140,"y":1700,"wires":[["b73ed76d.7bd3b8"]]},{"id":"b73ed76d.7bd3b8","type":"function","z":"afe7af4b.c2261","name":"message array","func":"var m_out=[]; //rray for message objects\nvar message=msg.payload;\nfor (i=0;i<3;i++){\n \n message=message+i; //add count to message\n var newmsg={payload:message,topic:msg.topic}\n m_out.push(newmsg);\n}\nreturn[m_out];\n","outputs":1,"noerr":0,"x":340,"y":1700,"wires":[["549f2f6c.0cc2a"]]},{"id":"549f2f6c.0cc2a","type":"debug","z":"afe7af4b.c2261","name":"","active":true,"tosidebar":true,"console":false,"tostatus":false,"complete":"false","x":530,"y":1700,"wires":[]}]