Bambams club
A personal project blog

Setting an Arduino Up to Read a Tipping Bucket Rain Gauge
1 Reply
This post is part of a series I’m doing on the automatic first flush system we use on our rainwater harvesting set-up. The tipping bucket rain gauge is the key external input that allows the system to do its job. Tipping bucket rain gauges use a funnel to direct water onto a see-saw of two side-by-side buckets. The buckets are sized so that a certain amount of water causes the see-saw to tip (usually .01 inches of precipitation collected by the funnel, an 8 inch diameter funnel collects about 8.2 mL of water per tip).
When the see-saw tips, a magnet swings along with it and past a magnetic switch that closes as the magnet passes over. When the switch closes it briefly completes the circuit and a pulse is sent down the output wire. The unpredictable, short nature of the pulse being sent down the wire makes it a bit hard to measure with an arduino that is doing lots of other things. What if the pulse comes and the arduino is busy doing something else? To solve this problem, I hooked the output of the tipping bucket gauge up to a flip-flop, a very simple combination of logic gates housed inside of an integrated circut (you can get one from radio shack for less than $1). The flip flop captures the pulse from the gauge, and in effect holds on to it by setting its own output high (to 5 volts). Even when the pulse from the rain gauge stops, the flip flop’s output remains high. This buys the arduino some time to check on the rain gauge when it gets around to it. The arduino sketch (program) is set to check the flip flop each cycle. If the line is high, it records that .01 inches of rain as fallen, adds that amount to the storm total, and then sends a pulse to the reset pin on the flip flop. When the reset pin on the flip flop is set high, it effectively lets go of the last tip and is then ready to record a new one. If we don’t want to miss any tips, we have to be sure the rest of our arduino sketch doesn’t take longer than the minimum time between tips. On most tipping bucket gauges, they don’t tip much faster than once per second. As long as your sketch loops faster than that, you should get a fairly accurate count from your gauge.

The basics of how the tipping bucket, flip flop, and arduino are hooked up
Here is the rain gauge reading subroutine from the sketch:
void rainTip () { if (digitalRead(rain_Gauge) == HIGH) { //if the flip flop has a tip recorded timeOfLastTip = now(); //timestamp the latest tip so we can calculate dry-times ++rainCountTips; //add to the storm total rainCountIn = rainCountTips * .01; //convert from tips to inches digitalWrite(rain_GaugeReset, HIGH); //send a pulse to reset the flip flop delay(600); //hold it for long enough to actually work digitalWrite(rain_GaugeReset, LOW); //set the reset pin low } // END of if } // END of subroutine
timer.setInterval(10L,rain); // set interval to read rain gauge
void rain(){
int buttonState = digitalRead(rainGauge);
// print out the state of the button:
// Serial.println(buttonState);
if(buttonState==LOW) // it is raining
{
rainAmount = .3; //amount of rain with each bucket tip in gauge
totalAmount += rainAmount; //running total of rain that has fallen
Blynk.virtualWrite(V13,totalAmount); // send rain amount to Blynk app
Serial.print("this is the total rain that has fallen ");
Serial.print(totalAmount);
Serial.println(" mm");
}
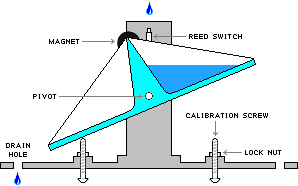
![Illustration of a rain gauge sensor [3].](https://www.researchgate.net/profile/Amit_Sheth/publication/228682193/figure/fig1/AS:301879679569920@1448985132034/Illustration-of-a-rain-gauge-sensor-3.png)
Illustration of a rain gauge sensor [3].
Source publication